Create a virtual keyboard that users can interact with on the webpage.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Virtual Keyboard</title>
<style>
body {
font-family: 'Arial', sans-serif;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
margin: 0;
background-color: #f4f4f4;
}
#keyboard {
display: grid;
grid-template-columns: repeat(10, 1fr);
gap: 5px;
max-width: 500px;
margin-bottom: 20px;
}
button {
padding: 10px;
font-size: 16px;
border: 1px solid #ccc;
background-color: #fff;
cursor: pointer;
}
#inputField {
font-size: 16px;
padding: 10px;
width: 300px;
margin-bottom: 20px;
}
</style>
</head>
<body>
<input type="text" id="inputField" readonly>
<div id="keyboard"></div>
<script>
document.addEventListener('DOMContentLoaded', function () {
const keyboard = document.getElementById('keyboard');
const inputField = document.getElementById('inputField');
const keys = [
'1', '2', '3', '4', '5', '6', '7', '8', '9', '0',
'Q', 'W', 'E', 'R', 'T', 'Y', 'U', 'I', 'O', 'P',
'A', 'S', 'D', 'F', 'G', 'H', 'J', 'K', 'L',
'Z', 'X', 'C', 'V', 'B', 'N', 'M',
'!', '@', '#', '$', '%', '^', '&', '*', '(', ')', '-',
'Tab', 'Shift', 'Control', 'Enter', 'Backspace',
'↑', '←', '↓', '→'
];
keys.forEach(key => {
const button = document.createElement('button');
button.textContent = key;
button.addEventListener('click', () => onKeyPress(key));
keyboard.appendChild(button);
});
function onKeyPress(key) {
switch (key) {
case 'Tab':
inputField.value += '\t';
break;
case 'Shift':
// Handle Shift key
break;
case 'Control':
// Handle Control key
break;
case 'Enter':
inputField.value += '\n';
break;
case 'Backspace':
inputField.value = inputField.value.slice(0, -1);
break;
case '↑':
// Handle up arrow key
break;
case '←':
// Handle left arrow key
break;
case '↓':
// Handle down arrow key
break;
case '→':
// Handle right arrow key
break;
default:
inputField.value += key;
break;
}
}
});
</script>
</body>
</html>
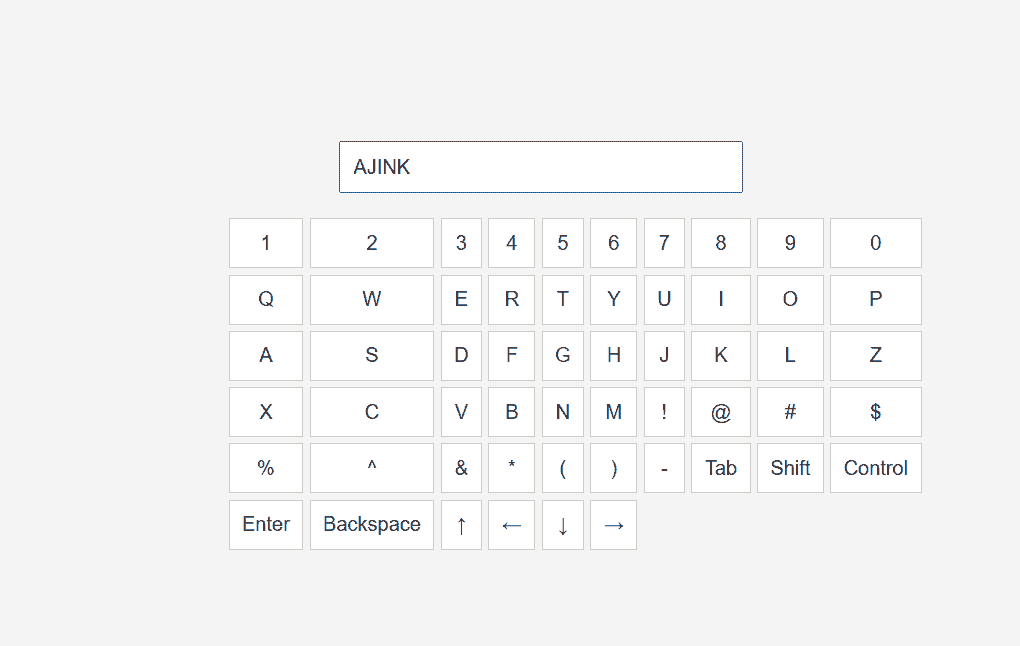