Blockchain Workshop Experience
Hey everyone, I’m Ajink, and in this blog, I’m going to share my learning experience from today’s workshop on blockchain organized by SHAIDS DMCE. Our speaker for the session was Dhruv Godambe.
Getting Started: Installation
The workshop began with the installation of Ganache and Truffle.
Installation Commands and Links
Ganache:
Ganache is a personal blockchain for Ethereum development you can use to deploy contracts, develop your applications, and run tests.
alternatively you can also use genache-cli
Truffle:
Truffle is a development environment, testing framework, and asset pipeline for blockchains using the Ethereum Virtual Machine (EVM).
Install Truffle globally using npm:
npm install -g truffle
Setting Up the Project
We started by creating a new folder and initializing a Truffle project:
mkdir blockchain
cd blockchain
truffle init
Creating a Solidity File
Next, we created a Solidity file named init.sol
in the contracts
folder created by Truffle. Here is the code:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract Init {
// states
uint counter;
// functions
// getter fn
function get_counter() public view returns (uint) {
return counter;
}
// setter fn
function set_counter() public {
counter = counter + 1;
}
}
Explanation of the Solidity Code
- State Variables: We have a
uint
variable namedcounter
. - Getter Function:
get_counter()
returns the current value ofcounter
. - Setter Function:
set_counter()
increments the value ofcounter
by 1.
Running Ganache and Configuring Truffle
We opened Ganache to start the local blockchain and then configured the truffle-config.js
file to uncomment the development environment:
development: {
host: "127.0.0.1", // Localhost (default: none)
port: 8545, // Standard Ethereum port (default: none)
network_id: "5777", // Any network (default: none)
}
Creating a Migration File
We created a migration file to deploy our contract in migrations folder with name 01_deploy_init.js :
const Init = artifacts.require("Init");
module.exports = function(deployer, network, accounts) {
deployer.deploy(Init)
.then(() => {
console.log("Init deployed at:", Init.address);
});
};
Deployed smart contract on local network
truffle compile
truffle migrate --network development
Interacting with Sepolia Testnet and Remix
After this, we learned how to interact with the Sepolia testnet using Remix.
Creating Vault.sol
We created another Solidity file named Vault.sol
:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract Vault {
mapping(address => uint) vaultBalances;
function deposit() public payable {
vaultBalances[msg.sender] += msg.value;
}
function getVaultBalance(address _user) public view returns (uint) {
return vaultBalances[_user];
}
function withdraw() public {
uint userBalance = vaultBalances[msg.sender];
require(userBalance > 0, "No balance to withdraw");
vaultBalances[msg.sender] = 0;
payable(msg.sender).transfer(userBalance);
}
}
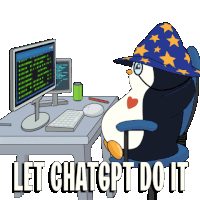
Explanation of the Solidity Code
- State Variables:
vaultBalances
keeps track of each user’s balance. - Deposit Function:
deposit()
allows users to add funds to the vault. - Get Balance Function:
getVaultBalance()
returns the balance of a specified user. - Withdraw Function:
withdraw()
allows users to withdraw their balance from the vault.
We connected with MetaMask, deployed the smart contract using Remix, and performed deposit, withdraw, and balance view functions.
Using Truffle with Sepolia
We then performed the same actions on our machine using Truffle with the Sepolia testnet, Alchemy, and our wallet’s private key. We edited the truffle-config.js
file:
sepolia: {
provider: () => new HDWalletProvider("your-private-key", `alchemy-endpoint`),
network_id: 11155111, // Sepolia's id
confirmations: 2, // # of confirmations to wait between deployments. (default: 0)
timeoutBlocks: 200, // # of blocks before a deployment times out (minimum/default: 50)
skipDryRun: true // Skip dry run before migrations? (default: false for public nets)
}
We then created Vault.sol
in the contracts
folder and edited the migration file to deploy Vault:
const Vault = artifacts.require("Vault");
module.exports = function(deployer, network, accounts) {
deployer.deploy(Vault)
.then(() => {
console.log("Vault deployed at:", Vault.address);
});
};
Deployment
We opened the terminal and ran the following command:
truffle migrate --network sepolia
We encountered an error related to HDWalletProvider, which we resolved by installing the package:
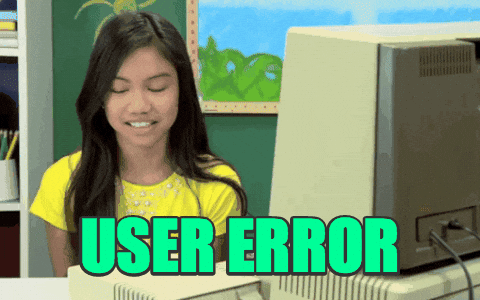
npm i @truffle/hdwallet-provider
We then re-ran the migration command:
truffle migrate --network sepolia
Our smart contract was successfully deployed!
Conclusion
Thanks for reading! This was Day 01 and Day 02 of our blockchain workshop experience. Stay tuned for more updates!
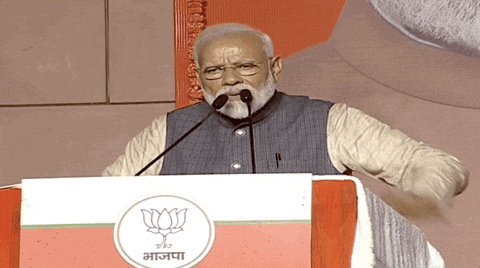