Hey , I am Ajink in this post we will create a virtual canvas where you can draw and paint using html5 and javascript !
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Virtual Canvas</title>
<style>
body {
font-family: 'Arial', sans-serif;
margin: 0;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
height: 100vh;
background-color: #f4f4f4;
}
#toolbox {
position: fixed;
top: 0;
left: 0;
width: 100%;
background-color: #fff;
padding: 10px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
z-index: 1000;
display: flex;
justify-content: space-between;
align-items: center;
}
canvas {
border: 1px solid #000;
margin-top: 60px; /* Adjusted to accommodate the fixed toolbox */
cursor: crosshair;
width: 100%; /* Make canvas responsive */
max-width: 800px; /* Limit canvas width for larger screens */
}
#colorPicker,
#brushSize,
#imageInput,
button {
margin-right: 10px;
padding: 5px 10px;
font-size: 14px;
cursor: pointer;
border: none;
border-radius: 5px;
}
#controls {
display: flex;
flex-wrap: wrap;
justify-content: flex-end;
align-items: center;
margin-top: 10px;
}
input[type="range"] {
width: 150px;
}
</style>
</head>
<body>
<div id="toolbox">
<div>
<input type="color" id="colorPicker" value="#000000">
<label for="brushSize">Brush Size:</label>
<input type="range" id="brushSize" min="1" max="20" value="5">
</div>
<div>
<button onclick="clearCanvas()">Clear</button>
<input type="file" id="imageInput" accept="image/*">
</div>
</div>
<canvas id="myCanvas" width="800" height="600"></canvas>
<script>
document.addEventListener('DOMContentLoaded', function () {
var canvas = document.getElementById('myCanvas');
var context = canvas.getContext('2d');
var painting = false;
var colorPicker = document.getElementById('colorPicker');
var brushSizeInput = document.getElementById('brushSize');
var imageInput = document.getElementById('imageInput');
canvas.addEventListener('mousedown', startPainting);
canvas.addEventListener('mouseup', stopPainting);
canvas.addEventListener('mousemove', paint);
colorPicker.addEventListener('input', setColor);
brushSizeInput.addEventListener('input', setBrushSize);
imageInput.addEventListener('change', pasteImage);
canvas.addEventListener('touchstart', function (e) {
startPainting(e.touches[0]);
});
canvas.addEventListener('touchend', stopPainting);
canvas.addEventListener('touchmove', function (e) {
paint(e.touches[0]);
e.preventDefault();
});
context.lineWidth = 5;
context.lineCap = 'round';
context.strokeStyle = '#000';
function startPainting(e) {
painting = true;
paint(e);
}
function stopPainting() {
painting = false;
context.beginPath();
}
function paint(e) {
if (!painting) return;
context.lineTo(e.clientX - canvas.offsetLeft, e.clientY - canvas.offsetTop);
context.stroke();
context.beginPath();
context.moveTo(e.clientX - canvas.offsetLeft, e.clientY - canvas.offsetTop);
}
function setColor() {
context.strokeStyle = colorPicker.value;
}
function setBrushSize() {
context.lineWidth = brushSizeInput.value;
}
function pasteImage(e) {
var file = e.target.files[0];
if (file) {
var reader = new FileReader();
reader.onload = function (event) {
var img = new Image();
img.onload = function () {
context.drawImage(img, 0, 0, canvas.width, canvas.height);
};
img.src = event.target.result;
};
reader.readAsDataURL(file);
}
}
function clearCanvas() {
context.clearRect(0, 0, canvas.width, canvas.height);
}
});
</script>
</body>
</html>
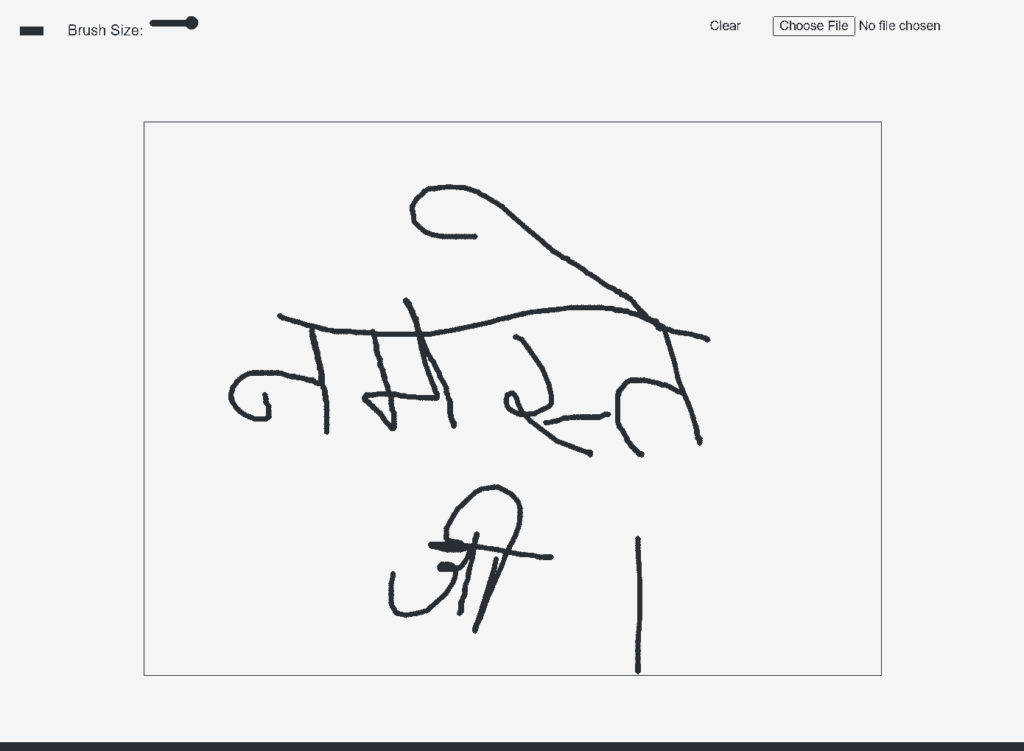